Display icon associated with an executableTag(s): Swing
First technique
import java.io.*; import javax.swing.*; public class IconExtract1 { public static void main(String[] args) throws Exception { String s = "c:/windows/regedit.exe"; File file = new File(s); // Get metadata and create an icon sun.awt.shell.ShellFolder sf = sun.awt.shell.ShellFolder.getShellFolder(file); Icon icon = new ImageIcon(sf.getIcon(true)); System.out.println("type = " + sf.getFolderType()); // show the icon JLabel ficon = new JLabel(s, icon, SwingConstants.LEFT); JFrame frame = new JFrame(); frame.getContentPane().add(ficon); frame.pack(); frame.setVisible(true); } }
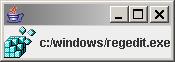
Second technique
import java.io.*; import javax.swing.*; import java.awt.*; import javax.swing.filechooser.FileSystemView; public class IconExtract2 { public static void main(String[] args) throws Exception { String s = "c:/windows/regedit.exe"; File file = new File(s); // Get metadata and create an icon Icon icon = FileSystemView.getFileSystemView().getSystemIcon(file); // show the icon JLabel ficon = new JLabel(s, icon, SwingConstants.LEFT); JFrame frame = new JFrame(); frame.getContentPane().add(ficon); frame.pack(); frame.setVisible(true); } }
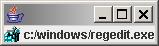
If you need more generic image, you use a JFileChooser and extract the image for a file or directory.
import java.io.*; import javax.swing.*; import java.awt.*; import javax.swing.filechooser.*; public class IconExtract3 { public static void main(String[] args) throws Exception { String s = "c:/windows/regedit.exe"; JFileChooser chooser = new JFileChooser(); File file = new File(s); Icon icon = chooser.getIcon(file); // show the icon JLabel ficon = new JLabel(s, icon, SwingConstants.LEFT); JFrame frame = new JFrame(); frame.getContentPane().add(ficon); frame.pack(); frame.setVisible(true); } }
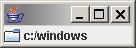
output for a file :
mail_outline
Send comment, question or suggestion to howto@rgagnon.com
Send comment, question or suggestion to howto@rgagnon.com